「gocuiのコンポーネントライブラリを作った話」という記事を読んでいて、rivo/tviewというライブラリを使うと、CUIをお手軽に作成できるようなので、お試しで使ってみました。
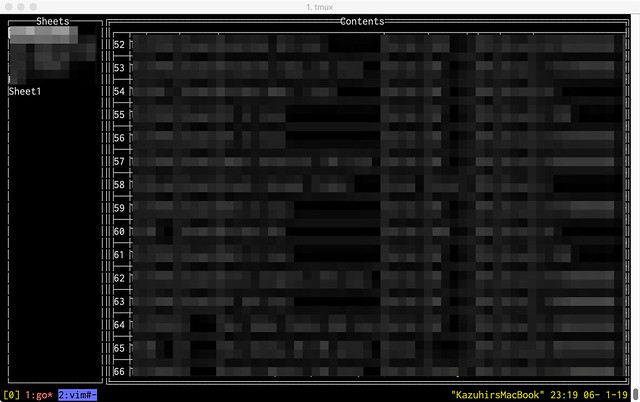
細々と説明していきます。
GolangでExcelファイル(.xlsx)を読み込むを参考にしましたよ。
こちらになります:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
| package main
import (
"fmt"
"os"
"strconv"
"github.com/gdamore/tcell"
"github.com/rivo/tview"
"github.com/tealeg/xlsx"
)
func main() {
app := tview.NewApplication()
// Generate Sheet List Instance
generateSheets := func() *tview.List {
result := tview.NewList().ShowSecondaryText(false)
result.SetBorder(true).SetTitle("Sheets")
return result
}
sheets := generateSheets()
// Generate Column Table Instance
tables := tview.NewTable().SetBorders(true)
tables.SetBorder(true).SetTitle("Contents")
// Create Excel instance
createExcelInstance := func(excelFileName string) *xlsx.File {
xlFile, err := xlsx.OpenFile(excelFileName)
if err != nil {
panic(err)
}
return xlFile
}
excel := createExcelInstance("./test.xlsx")
// Add Sheets to Sheet List
addSheets := func(sheets *tview.List, excelInstance *xlsx.File) {
for _, sheet := range excelInstance.Sheets {
tmp := sheet
sheets.AddItem(tmp.Name, "", 0, func() {
// Clear the table
tables.Clear()
for i, row := range tmp.Rows {
// Add row number:
tables.SetCellSimple(i, 0, strconv.Itoa(i+1))
for j, cell := range row.Cells {
tables.SetCellSimple(i, j+1, cell.String())
}
}
tables.ScrollToBeginning()
app.SetFocus(tables)
})
sheets.SetDoneFunc(func() {
app.Stop()
os.Exit(0)
})
tables.SetDoneFunc(func(key tcell.Key) {
switch key {
case tcell.KeyEscape:
tables.Clear()
app.SetFocus(sheets)
case tcell.KeyEnter:
// Press Enter to select the rows
tables.SetSelectable(true, false)
}
})
tables.SetSelectedFunc(func(row int, column int) {
for i := 0; i < tables.GetColumnCount(); i++ {
tables.GetCell(row, i).SetTextColor(tcell.ColorRed)
}
tables.SetSelectable(false, false)
})
}
}
addSheets(sheets, excel)
flex := tview.NewFlex().
AddItem(sheets, 0, 1, true).
AddItem(tables, 0, 5, false)
if err := app.SetRoot(flex, true).Run(); err != nil {
fmt.Printf("Error running application: %s\n", err)
}
}
|
お仕事で利用しているとあるExcelファイル、とても分析がし辛いフォーマットになっていて、それをいい感じにするツールを個人用に作成していたのですが、誰でも活用できるようにしていこうと思っています。Excelマクロは個人的に触りたくないので、これでどうにかしていこうと思っています。